Android/LiveData
Fragment - LiveData / LifeCycleOwner
${Harvey}
2022. 11. 22. 17:45
MainActivity.kt
// Fragment LiveData / viewLifecyclerOwner
// https://developer.android.google.cn/codelabs/kotlin-android-training-live-data/index.html?index=..%2F..android-kotlin-fundamentals&hl=IT#4
// https://developer.android.com/topic/libraries/view-binding
class MainActivity : AppCompatActivity() {
private lateinit var binding : ActivityMainBinding
val manager = supportFragmentManager
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
val view = binding.root
setContentView(view)
binding.btn1.setOnClickListener {
val transaction1 = manager.beginTransaction()
val fragment1 = BlankFragment1()
transaction1.replace(R.id.frameArea, fragment1)
transaction1.addToBackStack(null)
transaction1.commit()
}
BlankViewModel.kt
class BlankViewModel1 : ViewModel() {
private var _mutableCount = MutableLiveData(0)
val liveCount : LiveData<Int>
get() = _mutableCount
fun plusCountValue(){
_mutableCount.value = _mutableCount.value?.plus(1)
}
}
BlankFragment1.kt
class BlankFragment1 : Fragment() {
// ViewBinding + LiveData
private val TAG = "BlankFragment1"
private var _binding : FragmentBlank1Binding? = null
private val binding get() = _binding!!
private lateinit var viewModel: BlankViewModel1
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
}
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?,
): View? {
// Inflate the layout for this fragment
_binding = FragmentBlank1Binding.inflate(inflater, container, false)
val view = binding.root
viewModel = ViewModelProvider(this).get(BlankViewModel1::class.java)
return view
}
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
binding.btn1.setOnClickListener {
viewModel.plusCountValue()
}
viewModel.liveCount.observe(viewLifecycleOwner, Observer {
binding.text1.text = it.toString()
})
}
override fun onDestroyView() {
super.onDestroyView()
Log.d(TAG, "onDestroyView")
}
override fun onDestroy() {
super.onDestroy()
Log.d(TAG, "onDestroy")
}
}

Fragment에선 생명주기가 달라 생길 수 있는 오류들을 해결하기위해 viewLifecycleOwner를 사용해야한다.
BlankFragment2.kt
class BlankFragment2 : Fragment() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
}
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_blank2, container, false)
}
}
결과:
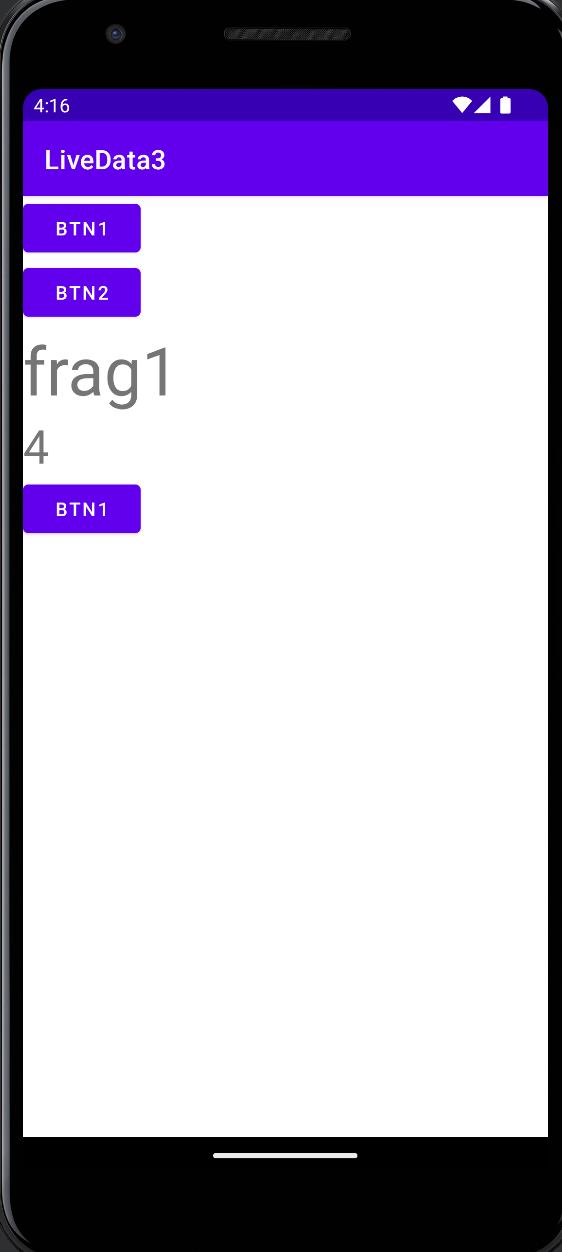